UI5, Babel and Gulp. The beginnings of a DevOps pipeline.
In this article I will be detailing a very simple DevOps pipeline that uses Babel.js and Gulp.js to transpile ECMAScript 6 to ES5, making it compatible with IE9!
Prerequisites: Node.js v8.11
npm install
Add the following dev dependencies to your project by either dropping them directly into your package.json
or by running npm install --save-dev ${package}
, where ${package}
is the package name e.g. @babel/core
.
{
"main": "index.html",
"devDependencies": {
"@babel/core": "^7.1.2",
"@babel/preset-env": "^7.1.0",
"babel-cli": "^6.26.0",
"gulp": "^3.9.1",
"gulp-babel": "^8.0.0",
"gulp-rename": "^1.4.0"
}
}
Once you have all of these packages successfully installed, run the command npm install
from the root directory.
Gulp tasks
Now create a gulpfile
(terminal command touch gulpfile.js
) at the root directory of your project and paste the below:
const gulp = require('gulp');
const rename = require('gulp-rename');
const babel = require('gulp-babel');
// default gulp task
gulp.task('default', () =>
// for each js file in the javascript folder
gulp.src('./javascript/*.js')
.pipe(babel({
// transpile ES6 to ES5
presets: ['@babel/preset-env']
}))
.pipe(rename((path) => {
// add file name suffix .controller to file
path.basename += '.controller';
}))
// save new js file to the controller folder
.pipe(gulp.dest('./controller'))
);
To run this build pipeline, run the gulp
command from the top-level directory of the project (where your gulpfile.js
sits).
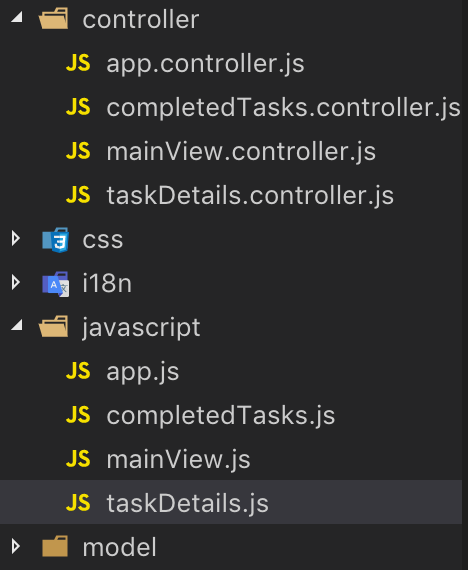
Example JavaScript in project
Currently this simple build script:
- Takes each javascript file from the
javascript
folder named. - Transpiles them to ES5 as per the preset-env (you can write your own preset)
- Adds the
.controller
suffix to the new file - Saves the new file in the
controller
folder
git and ui5 ignores
Your project .gitignore
should include:
/node_modules
/controller
Your project .Ui5RepositoryIgnore
should include:
/node_modules
/javascript
gulpfile.js
package.json
package-lock.json
Leave a comment
I hope that this tutorial has been helpful to you in modernising your UI5 workflow, I've setup an example repository here on GitHub. Leave a comment or tweet me with your thoughts and questions!